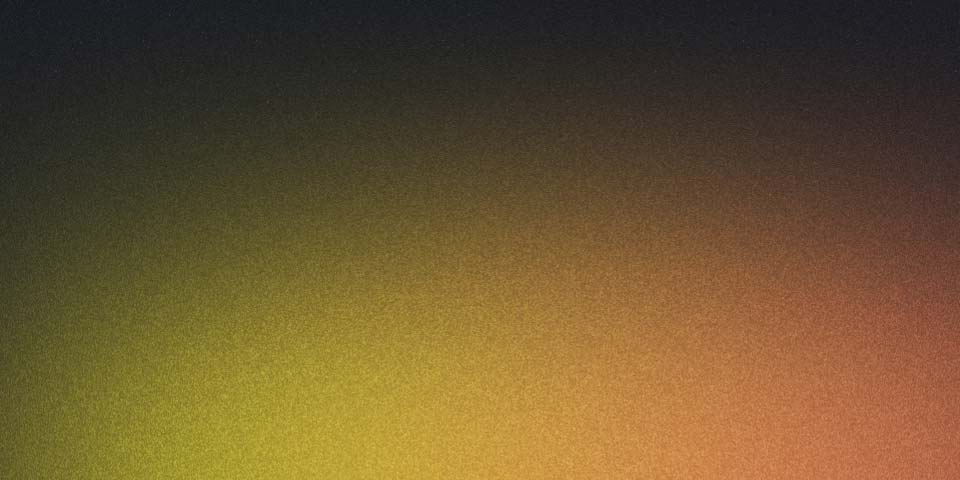
Setup a simple Vagrant box for Ansible examples
To setup a simple Vagrant box for Ansible experiments, we first need to make sure we have both VirtualBox and Vagrant installed. Both are simply a case of downloading the file and clicking to install. You’ll also need to be familiar with using the command line, if you’re not yet, you should check out Wes Bos’ Command Line Power User series.
Vagrant vs Docker
Both Vagrant and Docker are tools that take advantage of similar concepts and technologies with cross-over in their use.
Vagrant’s focus is in setting up Virtual Machines in a repeatable way that makes it quicker for Developers to get started on a project without needing to spend time configuring systems.
Docker builds immutable containers that are portable and can be combined in complex systems in a predictable way with a focus more on the needs of systems operating at scale.
Getting Started
You can create a new Vagrant box using the vagrant init command. For example to start with a plain Ubuntu 16.04 LTS Virtual Machine, just run vagrant init ubuntu/xenial64 to create the Vagrantfile and then start the boot process by running vagrant up. Once the box has started, you can connect to it via ssh by running vagrant ssh. When finished, you can stop the Virtual Machine with vagrant halt or delete it completely with vagrant destroy.
When you run vagrant init the resulting Vagrantfile has a huge number of configuration options commented out for example purposes, but there are only three lines that you need to do anything:
Vagrantfile
Vagrant.configure("2") do |config|
config.vm.box = "ubuntu/xenial64"
end
Adding Vagrantfile to git and what to .gitignore
When you are using Vagrant in a project managed with git you should add Vagrantfile to the repository. Once you start a Vagrant box it also creates some additional files that should never be added to your git repository. Vagrant runtime details are under .vagrant, and they should be added to .gitignore. Depending on the base box your use some additional logging such the ubuntu-xenial-16.04-cloudimg-console.logubuntu-xenial-16.04-cloudimg-console.log that the ubuntu/xenial64box creates.
.gitignore
# .gitignore
.vagrant
ubuntu-xenial-16.04-cloudimg-console.log
Adding more system resources to a Vagrant Box by configuring the provider
The provider is the software used by Vagrant to run your Virtual Machine. VirtualBox is the default and is free across platforms. As a rule, if you need to use another Vagrant provider you’ll probably know why, but for the vast majority of people the default VirtualBox provider is what you want.
config.vm.provider
config.vm.provider "virtualbox" do |v|
v.memory = 2048
v.cpus = 2
end
Vagrantfile
Vagrant.configure("2") do |config|
config.vm.box = "ubuntu/xenial64"
config.vm.provider "virtualbox" do |v|
v.memory = 2048
v.cpus = 2
end
end
You can also limit the amount of CPU consumption each core can use – Useful if you wanted to increase the number of cores available without risking tying up all your system resources.
config.vm.provider
config.vm.provider "virtualbox" do |v|
v.customize ["modifyvm", :id, "--cpuexecutioncap", "90"]
v.memory = 2048
v.cpus = 2
end
Vagrantfile
# Vagrantfile
Vagrant.configure("2") do |config|
config.vm.box = "ubuntu/xenial64"
config.vm.provider "virtualbox" do |v|
v.memory = 2048
v.cpus = 2
end
end
Configure Vagrant to provision Ansible
Now you have a Virtual Machine available, you could just log in with vagrant ssh and install the software you want to use manually. But as soon as you vagrant destroy the box, those changes will be lost so we put our system configuration into the provision step.
The provision step is run the first run vagrant up and a new box is installed. Next time you start the box, it will be skipped but you can run it again with vagrant provision.
config.vm.provision ‘shell’
config.vm.provision "shell", inline: <<-SHELL
apt-get update
apt-get install -y software-properties-common
apt-add-repository ppa:ansible/ansible
apt-get update
apt-get install -y ansible
SHELL
Vagrantfile
Vagrant.configure("2") do |config|
config.vm.box = "ubuntu/xenial64"
config.vm.provider "virtualbox" do |v|
v.memory = 2048
v.cpus = 2
end
config.vm.provision "shell", inline: <<-SHELL
apt-get update
apt-get install -y software-properties-common
apt-add-repository ppa:ansible/ansible
apt-get update
apt-get install -y ansible
SHELL
end